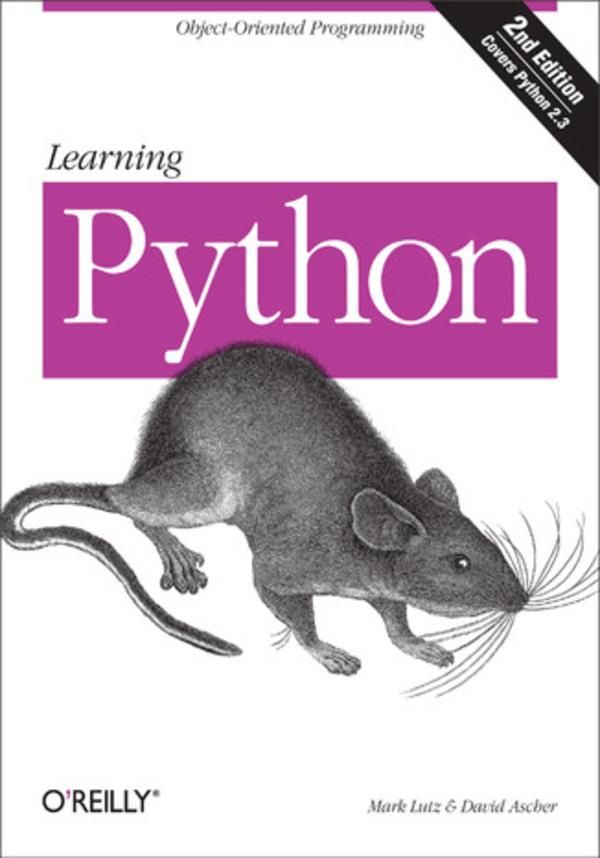
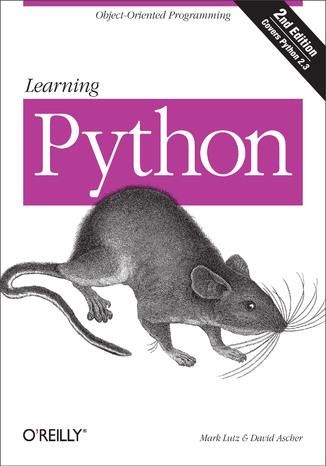
Learning Python. 2nd Edition (e-book)



Learning Python. 2nd Edition (e-book) - Najlepsze oferty
Learning Python. 2nd Edition (e-book) - Opis
Portable, powerful, and a breeze to use, Python is the popular open source object-oriented programming language used for both standalone programs and scripting applications. Python is considered easy to learn, but there's no quicker way to mastery of the language than learning from an expert teacher. This edition of Learning Python puts you in the hands of two expert teachers, Mark Lutz and David Ascher, whose friendly, well-structured prose has guided many a programmer to proficiency with the language. Learning Python, Second Edition, offers programmers a comprehensive learning tool for Python and object-oriented programming. Thoroughly updated for the numerous language and class presentation changes that have taken place since the release of the first edition in 1999, this guide introduces the basic elements of the latest release of Python 2.3 and covers new features, such as list comprehensions, nested scopes, and iterators/generators. Beyond language features, this edition of Learning Python also includes new context for less-experienced programmers, including fresh overviews of object-oriented programming and dynamic typing, new discussions of program launch and configuration options, new coverage of documentation sources, and more. There are also new use cases throughout to make the application of language features more concrete. The first part of Learning Python gives programmers all the information they'll need to understand and construct programs in the Python language, including types, operators, statements, classes, functions, modules and exceptions. The (...) więcej authors then present more advanced material, showing how Python performs common tasks by offering real applications and the libraries available for those applications. Each chapter ends with a series of exercises that will test your Python skills and measure your understanding.Learning Python, Second Edition is a self-paced book that allows readers to focus on the core Python language in depth. As you work through the book, you'll gain a deep and complete understanding of the Python language that will help you to understand the larger application-level examples that you'll encounter on your own. If you're interested in learning Python--and want to do so quickly and efficiently--then Learning Python, Second Edition is your best choice. Spis treści:Learning Python, 2nd Edition
SPECIAL OFFER: Upgrade this ebook with OReilly
A Note Regarding Supplemental Files
Preface
About This Second Edition
Prerequisites
This Books Scope
This Book's Style and Structure
Core Language
Outer Layers
Appendixes
Book Updates
Font Conventions
About the Programs in This Book
Using Code Examples
How to Contact Us
Acknowledgments
Mark Also Says:
David Also Says:
I. Getting Started
1. A Python Q&A Session
1.1. Why Do People Use Python?
1.1.1. Software Quality
1.1.2. Developer Productivity
1.2. Is Python a Scripting Language?
1.3. Okay, But What's the Downside?
1.4. Who Uses Python Today?
1.5. What Can I Do with Python?
1.5.1. Systems Programming
1.5.2. GUIs
1.5.3. Internet Scripting
1.5.4. Component Integration
1.5.5. Database Programming
1.5.6. Rapid Prototyping
1.5.7. Numeric Programming
1.5.8. Gaming, Images, AI, XML, and More
1.6. What Are Python's Technical Strengths?
1.6.1. It's Object-Oriented
1.6.2. It's Free
1.6.3. It's Portable
1.6.4. It's Powerful
1.6.5. It's Mixable
1.6.6. It's Easy to Use
1.6.7. It's Easy to Learn
1.7. How Does Python Stack Up to Language X?
2. How Python Runs Programs
2.1. Introducing the Python Interpreter
2.2. Program Execution
2.2.1. The Programmer's View
2.2.2. Python's View
2.2.2.1. Byte code compilation
2.2.2.2. Python Virtual Machine (PVM)
2.2.2.3. Performance implications
2.2.2.4. Development implications
2.3. Execution Model Variations
2.3.1. Python Implementation Alternatives
2.3.1.1. CPython
2.3.1.2. Jython
2.3.1.3. Python.NET
2.3.2. The Psyco Just-in-Time Compiler
2.3.3. Frozen Binaries
2.3.4. Future Possibilities?
3. How You Run Programs
3.1. Interactive Coding
3.1.1. Using the Interactive Prompt
3.2. System Command Lines and Files
3.2.1. Using Command Lines and Files
3.2.2. Unix Executable Scripts (#!)
3.3. Clicking Windows File Icons
3.3.1. Clicking Icons on Windows
3.3.2. The raw_input Trick
3.3.3. Other Icon Click Limitations
3.4. Module Imports and Reloads
3.4.1. The Grander Module Story: Attributes
3.4.2. Import and Reload Usage Notes
3.5. The IDLE User Interface
3.5.1. IDLE Basics
3.5.2. Using IDLE
3.6. Other IDEs
3.7. Embedding Calls
3.8. Frozen Binary Executables
3.9. Text Editor Launch Options
3.10. Other Launch Options
3.11. Future Possibilities?
3.12. Which Option Should I Use?
3.13. Part I Exercises
II. Types and Operations
4. Numbers
4.1. Python Program Structure
4.2. Why Use Built-in Types?
4.3. Numbers
4.3.1. Number Literals
4.3.2. Built-in Tools and Extensions
4.4. Python Expression Operators
4.4.1. Mixed Operators: Operator Precedence
4.4.2. Parentheses Group Subexpressions
4.4.3. Mixed Types: Converted Up
4.4.4. Preview: Operator Overloading
4.5. Numbers in Action
4.5.1. Basic Operations and Variables
4.5.2. Numeric Representation
4.5.3. Division: Classic, Floor, and True
4.5.4. B itwise Operations
4.5.5. L ong Integers
4.5.6. Complex Numbers
4.5.7. Hexadecimal and Octal Notation
4.5.8. Other Numeric Tools
4.6. The Dynamic Typing Interlude
4.6.1. How Assignments Work
4.6.2. References and Changeable Objects
4.6.3. References and Garbage Collection
5. Strings
5.1. String Literals
5.1.1. Single- and Double-Quoted Strings Are the Same
5.1.2. Escape Sequences Code Special Bytes
5.1.3. Raw Strings Suppress Escapes
5.1.4. Triple Quotes Code Multiline Block Strings
5.1.5. Unicode Strings Encode Larger Character Sets
5.2. Strings in Action
5.2.1. Basic Operations
5.2.2. Indexing and Slicing
5.2.2.1. I ndexing (S[i]) fetches components at offsets
5.2.2.2. S licing (S[i:j]) extracts contiguous sections of a sequence
5.2.3. String Conversion Tools
5.2.4. C hanging Strings
5.3. String Formatting
5.3.1. Advanced String Formatting
5.4. String Methods
5.4.1. String Method Examples: Changing Strings
5.4.2. String Method Examples: Parsing Text
5.4.3. The Original Module
5.5. General Type Categories
5.5.1. Types Share Operation Sets by Categories
5.5.2. Mutable Types Can Be Changed in-Place
6. Lists and Dictionaries
6.1. Lists
6.2. Lists in Action
6.2.1. Basic List Operations
6.2.2. In dexing, Slicing, and Matrixes
6.2.3. Changing Lists in-Place
6.2.3.1. Index and slice assignment
6.2.3.2. List method calls
6.3. Dictionaries
6.4. Dictionaries in Action
6.4.1. Basic Dictionary Operations
6.4.2. Changing Dictionaries in-Place
6.4.3. More Dictionary Methods
6.4.4. A Languages Table
6.4.5. Dictionary Usage Notes
6.4.5.1. Using dictionaries to simulate flexible lists
6.4.5.2. Using dictionaries for sparse data structures
6.4.5.3. Using dictionaries as "records"
7. Tuples, Files, and Everything Else
7.1. Tuples
7.1.1. Why Lists and Tuples?
7.2. Files
7.2.1. Files in Action
7.3. Type Categories Revisited
7.4. Object Generality
7.5. References Versus Copies
7.6. Comparisons, Equality, and Truth
7.7. Python's Type Hierarchies
7.8. Other Types in Python
7.9. Built-in Type Gotchas
7.9.1. Assignment Creates References, Not Copies
7.9.2. Repetition Adds One-Level Deep
7.9.2.1. Solutions
7.9.3. Cyclic Data Structures
7.9.4. Immutable Types Can't Be Changed in-Place
7.10. Part II Exercises
III. Statements and Syntax
8. Assignment, Expressions, and Print
8.1. Assignment Statements
8.1.1. Variable Name Rules
8.1.1.1. Naming conventions
8.1.1.2. Names have no type, but objects do
8.1.2. Augmented Assignment Statements
8.2. Expression Statements
8.3. Print Statements
8.3.1. The Python "Hello World" Program
8.3.2. Redirecting the Output Stream
8.3.2.1. The printfile extension
9. if Tests
9.1. if Statements
9.1.1. General Format
9.1.2. Examples
9.1.2.1. Multiway branching
9.2. Python Syntax Rules
9.2.1. Block Delimiters
9.2.2. Statement Delimiters
9.2.3. A Few Special Cases
9.3. Truth Tests
10. while and for Loops
10.1. while Loops
10.1.1. General Format
10.1.2. Examples
10.2. break, continue, pass, and the Loop else
10.2.1. General Loop Format
10.2.2. Examples
10.2.3. More on the Loop else
10.3. for Loops
10.3.1. General Format
10.3.2. Examples
10.4. Loop Variations
10.4.1. Counter Loops: range
10.4.2. Nonexhaustive Traversals: range
10.4.3. Changing Lists: range
10.4.4. Parallel Traversals: zip and map
10.4.5. Dictionary Construction with zip
11. Documenting Python Code
11.1. The Python Documentation Interlude
11.1.1. Documentation Sources
11.1.2. # Comments
11.1.3. The dir Function
11.1.4. Docstrings: __doc__
11.1.4.1. User-defined docstrings
11.1.4.2. Docstring standards
11.1.4.3. Built-in docstrings
11.1.5. PyDoc: The help Function
11.1.6. PyDoc: HTML Reports
11.1.7. Standard Manual Set
11.1.8. Web Resources
11.1.9. Published Books
11.2. Common Coding Gotchas
11.3. Part III Exercises
IV. Functions
12. Function Basics
12.1. Why Use Functions?
12.2. Coding Functions
12.2.1. def Statements
12.2.2. def Executes at Runtime
12.3. A First Example: Definitions and Calls
12.3.1. Definition
12.3.2. Calls
12.3.3. Polymorphism in Python
12.4. A Second Example: Intersecting Sequences
12.4.1. Definition
12.4.2. Calls
12.4.3. Polymorphism Revisited
12.4.4. Local Variables
13. Scopes and Arguments
13.1. Scope Rules
13.1.1. Python Scope Basics
13.1.2. Name Resolution: The LEGB Rule
13.1.3. Scope Example
13.1.4. The Built-in Scope
13.2. The global Statement
13.3. Scopes and Nested Functions
13.3.1. Nested Scope Details
13.3.2. Nested Scope Examples
13.4. Passing Arguments
13.4.1. Arguments and Shared References
13.4.2. Avoiding Mutable Argument Changes
13.4.3. Simulating Output Parameters
13.5. Special Argument Matching Modes
13.5.1. Keyword and Default Examples
13.5.2. Arbitrary Arguments Examples
13.5.3. Combining Keywords and Defaults
13.5.4. The min Wakeup Call
13.5.4.1. Full credit
13.5.4.2. Bonus points
13.5.4.3. The punch line
13.5.5. A More Useful Example: General Set Functions
13.5.6. Argument Matching: The Gritty Details
14. Advanced Function Topics
14.1. Anonymous Functions: lambda
14.1.1. lambda Expressions
14.1.2. Why lambda?
14.1.3. How (Not) to Obfuscate Your Python Code
14.1.4. Nested lambdas and Scopes
14.2. Applying Functions to Arguments
14.2.1. The apply Built-in
14.2.1.1. Passing keyword arguments
14.2.2. Apply-Like Call Syntax
14.3. Mapping Functions Over Sequences
14.4. Functional Programming Tools
14.5. List Comprehensions
14.5.1. List Comprehension Basics
14.5.2. Adding Tests and Nested Loops
14.5.3. Comprehending List Comprehensions
14.6. Generators and Iterators
14.6.1. Generator Example
14.6.2. Iterators and Built-in Types
14.7. Function Design Concepts
14.7.1. Functions Are Objects: Indirect Calls
14.8. Function Gotchas
14.8.1. Local Names Are Detected Statically
14.8.2. Defaults and Mutable Objects
14.8.3. Functions Without Returns
14.9. Part IV Exercises
V. Modules
15. Modules: The Big Picture
15.1. Why Use Modules?
15.2. Python Program Architecture
15.2.1. How to Structure a Program
15.2.2. Imports and Attributes
15.2.3. Standard Library Modules
15.3. How Imports Work
15.3.1. 1. Find It
15.3.1.1. The module search path
15.3.1.2. The sys.path list
15.3.1.3. Module file selection
15.3.2. 2. Compile It (Maybe)
15.3.3. 3. Run It
16. Module Coding Basics
16.1. Module Creation
16.2. Module Usage
16.2.1. The import Statement
16.2.2. The from statement
16.2.3. The from * statement
16.2.4. Imports Happen Only Once
16.2.5. import and from Are Assignments
16.2.6. Cross-File Name Changes
16.2.7. import and from Equivalence
16.3. Module Namespaces
16.3.1. Files Generate Namespaces
16.3.2. Attribute Name Qualification
16.3.3. Imports Versus Scopes
16.3.4. Namespace Nesting
16.4. Reloading Modules
16.4.1. Reload Basics
16.4.2. Reload Example
17. Module Packages
17.1. Package Import Basics
17.1.1. Packages and Search Path Settings
17.1.2. Package __init__.py Files
17.2. Package Import Example
17.2.1. from Versus import with Packages
17.3. Why Use Package Imports?
17.4. A Tale of Three Systems
18. Advanced Module Topics
18.1. Data Hiding in Modules
18.1.1. Minimizing from* damage: _X and __all__
18.2. Enabling Future Language Features
18.3. Mixed Usage Modes: __name__ and __main__
18.4. Changing the Module Search Path
18.5. The import as Extension
18.6. Module Design Concepts
18.6.1. Modules Are Objects: Metaprograms
18.7. Module Gotchas
18.7.1. Importing Modules by Name String
18.7.2. from Copies Names but Doesn't Link
18.7.3. Statement Order Matters in Top-Level Code
18.7.4. Recursive "from" Imports May Not Work
18.7.5. reload May Not Impact from Imports
18.7.6. reload and from and Interactive Testing
18.7.7. reload Isn't Applied Transitively
18.8. Part V Exercises
VI. Classes and OOP
19. OOP: The Big Picture
19.1. Why Use Classes?
19.2. OOP from 30,000 Feet
19.2.1. Attribute Inheritance Search
19.2.2. Coding Class Trees
19.2.3. OOP Is About Code Reuse
20. Class Coding Basics
20.1. Classes Generate Multiple Instance Objects
20.1.1. Class Objects Provide Default Behavior
20.1.2. Instance Objects Are Concrete Items
20.1.3. A First Example
20.2. Classes Are Customized by Inheritance
20.2.1. A Second Example
20.2.2. Classes and Modules
20.3. Classes Can Intercept Python Operators
20.3.1. A Third Example
20.3.2. Why Operator Overloading?
21. Class Coding Details
21.1. The Class Statement
21.1.1. General Form
21.1.2. Example
21.2. Methods
21.2.1. Example
21.2.2. Calling Superclass Constructors
21.2.3. Other Method Call Possibilities
21.3. Inheritance
21.3.1. Attribute Tree Construction
21.3.2. Specializing Inherited Methods
21.3.3. Class Interface Techniques
21.3.4. Abstract Superclasses
21.4. Operator Overloading
21.4.1. Common Operator Overloading Methods
21.4.2. __getitem__ Intercepts Index References
21.4.3. __getitem__ and __iter__ Implement Iteration
21.4.3.1. User-defined iterators
21.4.4. __getattr__ and __setattr__ Catch Attribute References
21.4.5. __repr__ and __str__Return String Representations
21.4.6. __radd__ Handles Right-Side Addition
21.4.7. __call__ Intercepts Calls
21.4.8. __del__ Is a Destructor
21.5. Namespaces: The Whole Story
21.5.1. Unqualified Names: Global Unless Assigned
21.5.2. Qualified Names: Object Namespaces
21.5.3. Assignments Classify Names
21.5.4. Namespace Dictionaries
21.5.5. Namespace Links
22. Designing with Classes
22.1. Python and OOP
22.1.1. Overloading by Call Signatures (or Not)
22.2. Classes as Records
22.3. OOP and Inheritance: "is-a" Relationships
22.4. OOP and Composition: "has-a" Relationships
22.4.1. Stream Processors Revisited
22.5. OOP and Delegation
22.6. Multiple Inheritance
22.7. Classes Are Objects: Generic Object Factories
22.7.1. Why Factories?
22.8. Methods Are Objects: Bound or Unbound
22.9. Documentation Strings Revisited
22.10. Classes Versus Modules
23. Advanced Class Topics
23.1. Extending Built-in Types
23.1.1. Extending Types by Embedding
23.1.2. Extending Types by Subclassing
23.2. Pseudo-Private Class Attributes
23.2.1. Name Mangling Overview
23.2.2. Why Use Pseudo-Private Attributes?
23.3. "New Style" Classes in Python 2.2
23.3.1. Diamond Inheritance Change
23.3.1.1. Diamond inheritance example
23.3.1.2. Explicit conflict resolution
23.3.2. Other New Style Class Extensions
23.3.2.1. Static and class methods
23.3.2.2. Instance slots
23.3.2.3. Class properties
23.3.2.4. New __getattribute__ overload method
23.4. Class Gotchas
23.4.1. Changing Class Attributes Can Have Side Effects
23.4.2. Multiple Inheritance: Order Matters
23.4.3. Class Function Attributes Are Special: Static Methods
23.4.3.1. Solution (prior to 2.2, and in 2.2 normally)
23.4.3.2. Static and class methods in Python 2.2
23.4.4. Methods, Classes, and Nested Scopes
23.4.5. Overwrapping-itis
23.5. Part VI Exercises
VII. Exceptions and Tools
24. Exception Basics
24.1. Why Use Exceptions?
24.1.1. Exception Roles
24.2. Exception Handling: The Short Story
24.3. The try/except/else Statement
24.3.1. Try Statement Clauses
24.3.2. The try/else Clause
24.3.3. Example: Default Behavior
24.3.4. Example: Catching Built-in Exceptions
24.4. The try/finally Statement
24.4.1. Example: Coding Termination Actions with try/finally
24.5. The raise Statement
24.5.1. Example: Raising and Catching User-Defined Exceptions
24.5.2. Example: Passing Extra Data with raise
24.5.3. Example: Propagating Exceptions with raise
24.6. The assert Statement
24.6.1. Example: Trapping Constraints (but Not Errors)
25. Exception Objects
25.1. String-Based Exceptions
25.2. Class-Based Exceptions
25.2.1. Class Exception Example
25.2.2. Why Class Exceptions?
25.2.3. Built-in Exception Classes
25.2.4. Specifying Exception Text
25.2.5. Sending Extra Data in Instances
25.2.5.1. Example: extra data with classes and strings
25.3. General raise Statement Forms
26. Designing with Exceptions
26.1. Nesting Exception Handlers
26.1.1. Example: Control-Flow Nesting
26.1.2. Example: Syntactic Nesting
26.2. Exception Idioms
26.2.1. Exceptions Aren't Always Errors
26.2.2. Functions Signal Conditions with raise
26.2.3. Debugging with Outer try Statements
26.2.4. Running in-Process Tests
26.3. Exception Design Tips
26.3.1. What Should Be Wrapped
26.3.2. Catching Too Much
26.3.3. Catching Too Little
26.4. Exception Gotchas
26.4.1. String Exceptions Match by Identity, Not Value
26.4.2. Catching the Wrong Thing
26.5. Core Language Summary
26.5.1. The Python Toolset
26.5.2. Development Tools for Larger Projects
26.6. Part VII Exercises
VIII. The Outer Layers
27. Common Tasks in Python
27.1. Exploring on Your Own
27.2. Conversions, Numbers, and Comparisons
27.3. Manipulating Strings
27.3.1. The string Module
27.3.2. Complicated String Matches with Regular Expressions
27.3.2.1. A real regular expression problem
27.4. Data Structure Manipulations
27.4.1. Making Copies
27.4.1.1. The copy module
27.4.2. Sorting
27.4.3. Randomizing
27.4.4. Making New Data Structures
27.4.5. Making New Lists and Dictionaries
27.5. Manipulating Files and Directories
27.5.1. The os and os.path Modules
27.5.2. Copying Files and Directories: The shutil Module
27.5.3. Filenames and Directories
27.5.4. Matching Sets of Files
27.5.5. Using Temporary Files
27.5.6. Modifying Input and Outputs
27.5.7. Using Standard I/O to Process Files
27.5.7.1. Finding all lines that start with a #
27.5.7.2. Extracting the fourth column of a file (where columns are defined by whitespace)
27.5.7.3. Extracting the fourth column of a file, where columns are separated by colons, and making it lowercase
27.5.7.4. Printing the first 10 lines, the last 10 lines, and every other line
27.5.7.5. Counting the number of times the word "Python" occurs in a file
27.5.7.6. Changing a list of columns into a list of rows
27.5.7.7. Choosing chunk sizes
27.5.8. Doing Something to a Set of Files Specified on the Command Line
27.5.9. Processing Each Line of One or More Files
27.5.10. Dealing with Binary Data: The struct Module
27.6. Internet-Related Modules
27.6.1. The Common Gateway Interface: The cgi Module
27.6.2. Manipulating URLs: The urllib and urlparse Modules
27.6.3. Specific Internet Protocols
27.6.4. Processing Internet Data
27.6.5. XML Processing
27.7. Executing Programs
27.8. Debugging, Testing, Timing, Profiling
27.8.1. Debugging with pdb
27.8.2. Testing with unittest
27.8.3. Timing
27.9. Exercises
28. Frameworks
28.1. An Automated Complaint System
28.1.1. Excerpt from the HTML File
28.2. Interfacing with COM: Cheap Public Relations
28.3. A Tkinter-Based GUI Editor for Managing Form Data
28.3.1. The Main Program
28.3.2. The Form Editor
28.3.3. Design Considerations
28.4. Jython: The Felicitous Union of Python and Java
28.4.1. Jython Gives Python Programmers Access to Java Libraries
28.4.2. Jython as a Java Scripting Language
28.4.3. A Real Jython/Swing Application: grapher.py
28.5. Exercises
29. Python Resources
29.1. Layers of Community
29.1.1. The Core
29.1.2. Local User Groups
29.1.3. Conferences and Workshops
29.1.4. Where to Get Help
29.1.4.1. Python-help
29.1.4.2. Python-tutor
29.1.4.3. Python-list
29.1.5. Special Interest Groups
29.1.6. python-dev
29.1.7. News Sources
29.2. The Process
29.3. Services and Products
29.4. The Legal Framework: The Python Software Foundation
29.5. Software
29.5.1. Search the Web
29.5.2. Search the Mailing List Archives
29.5.3. Look in the Vaults of Parnassus
29.5.4. Check the Python Package Index (PyPI)
29.5.5. Look in the Python Cookbook
29.6. Popular Third-Party Software
29.6.1. Interfaces to Windows and the MacOS
29.6.1.1. Windows
29.6.1.2. Macintosh
29.6.2. Special-Purpose Libraries
29.6.2.1. Scientific computing libraries
29.6.2.2. Relational database interfaces
29.6.2.3. Graphics libraries
29.6.2.3.1. Python Imaging Library (PIL)
29.6.2.3.2. PyGame and PyOpenGL
29.6.3. Interfaces to GUI Toolkits
29.6.4. Interfaces to C/C++/Fortran
29.6.4.1. SWIG and f2py
29.6.4.2. CXX and Boost::Python
29.6.4.3. Pyrex, Weave, and Psyco
29.6.4.4. ctypes
29.6.5. Little Gems
29.6.6. Packaging Tools
29.6.6.1. Installer
29.6.6.2. py2exe
29.7. Web Application Frameworks
29.7.1. Zope
29.7.2. Twisted
29.7.3. Quixote
29.7.4. Webware, Spyce, and More
29.8. Tools for Python Developers
IX. Appendixes
A. Installation and Configuration
A.1. Installing the Python Interpreter
A.1.1. Where to Get Python
A.1.2. Installation Steps
A.1.3. Configuration Steps
A.1.3.1. Python environment variables
A.1.4. How to Set Configuration Options
A.1.4.1. UNIX/Linux shell variables
A.1.4.2. DOS variables (Windows)
A.1.4.3. Other Windows options
A.1.4.4. Path files
B. Solutions to Exercises
B.1. Part I
B.2. Part II
B.3. Part III
B.4. Part IV
B.5. Part V
B.6. Part VI
B.7. Part VII
B.8. Part VIII
B.8.1. Chapter 27
B.8.2. Chapter 28
About the Authors
Colophon
SPECIAL OFFER: Upgrade this ebook with OReilly mniej
Learning Python. 2nd Edition (e-book) - Opinie i recenzje
Na liście znajdują się opinie, które zostały zweryfikowane (potwierdzone zakupem) i oznaczone są one zielonym znakiem Zaufanych Opinii. Opinie niezweryfikowane nie posiadają wskazanego oznaczenia.