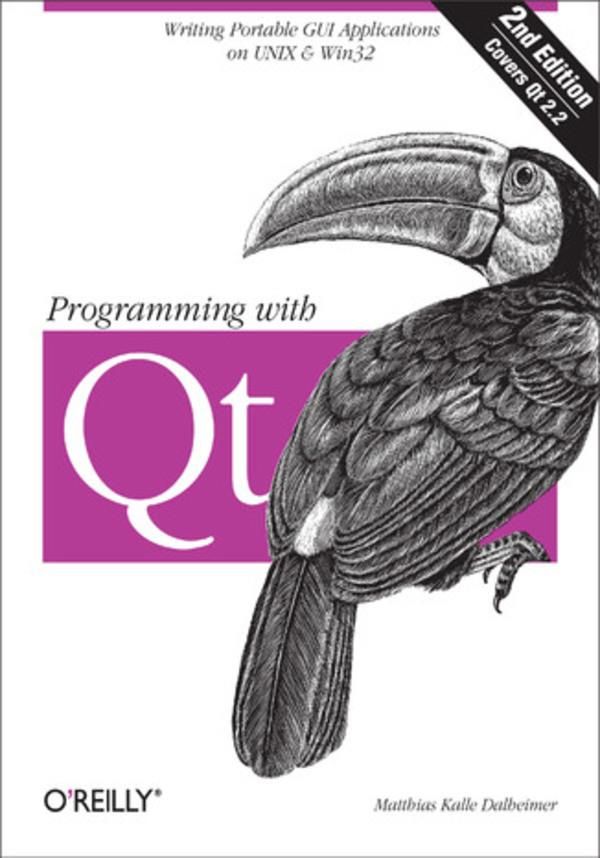
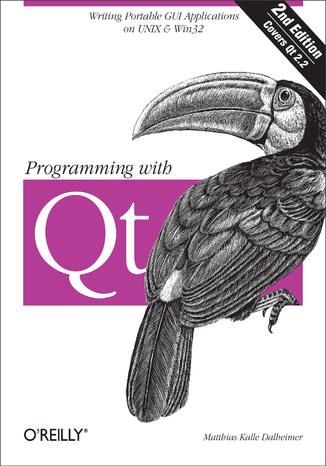
Programming with Qt. Writing Portable GUI applications on Unix and Win32. 2nd Edition (e-book)



Programming with Qt. Writing Portable GUI applications on Unix and Win32. 2nd Edition (e-book) - Najlepsze oferty
Programming with Qt. Writing Portable GUI applications on Unix and Win32. 2nd Edition (e-book) - Opis
The popular open source KDE desktop environment for Unix was built with Qt, a C++ class library for writing GUI applications that run on Unix, Linux, Windows 95/98, Windows 2000, and Windows NT platforms. Qt emulates the look and feel of Motif, but is much easier to use. Best of all, after you have written an application with Qt, all you have to do is recompile it to have a version that works on Windows. Qt also emulates the look and feel of Windows, so your users get native-looking interfaces.Platform independence is not the only benefit. Qt is flexible and highly optimized. You'll find that you need to write very little, if any, platform-dependent code because Qt already has what you need. And Qt is free for open source and Linux development.Although programming with Qt is straightforward and feels natural once you get the hang of it, the learning curve can be steep. Qt comes with excellent reference documentation, but beginners often find the included tutorial is not enough to really get started with Qt. That's whereProgramming with Qt steps in. You'll learn how to program in Qt as the book guides you through the steps of writing a simple paint application. Exercises with fully worked out answers help you deepen your understanding of the topics. The book presents all of the GUI elements in Qt, along with advice about when and how to use them, so you can make full use of the toolkit. For seasoned Qt programmers, there's also lots of information on advanced 2D transformations, drag-and-drop, writing custom image file filters, networking with the new Qt Network Extension, (...) więcej XML processing, Unicode handling, and more.Programming with Qt helps you get the most out of this powerful, easy-to-use, cross-platform toolkit. It's been completely updated for Qt Version 3.0 and includes entirely new information on rich text, Unicode/double byte characters, internationalization, and network programming. Spis treści:Programming with Qt, 2nd Edition
SPECIAL OFFER: Upgrade this ebook with OReilly
A Note Regarding Supplemental Files
Preface
A Productive Weekend
What You Should Know
Organization of This Book
Conventions Used in This Book
Comments and Questions
Acknowledgments
1. Introduction
Why GUI Toolkits?
Why Portability?
Why Qt?
Implementing Cross-Platform GUI Libraries
API Layering
API Emulation
GUI Emulation
Acquiring Qt
Qt Editions
How Free Is Qt?
Compiling and Installing Qt
Installing Qt or Qt/Embedded on Unix or MacOS X systems
Compiling and Installing Qt on Windows
C++ as Used by Qt
Getting Help
2. First Steps in Qt Programming
Hello, world!
Exercises
Using the Qt Reference Documentation
Exercises
Adding an Exit Button
Exercises
Introduction to Signals and Slots
The Problem of Callback Functions
A New Approach
Signals and Slots in Qt
Another Example of Signals and Slots
Running moc
Exercises
Event Handling and Simple Drawings with QPainter
Exercises
3. Learning More About Qt
Adding Menus
Exercises
Adding a Scrolled View
Exercises
Adding a Context Menu
Exercise
File I/O
Exercises
4. A Guided Tour Through the Simple Widgets
General Widget Parameters
Widget Styles
Buttons
Push Buttons
Radio Buttons and Checkboxes
Selection Widgets
List Boxes
Combo Boxes
Widgets for Bounded-Range Input
Sliders
Dials
Spin Boxes
Widgets for Entering Date and Time
Scrollbars
Menu-Related Widgets
Arrangers
Frames
Group Boxes
Splitters
Widget Stacks
Size Grips
Tab-Related Widgets
Text-Entry Fields
Labels
Simple Labels
QLCDNumber
Widgets for the Office
The Main Window
Toolbars
Status Bars
Multiple Document Interface (MDI)
Docking Windows
Tooltips and Whats This Windows
Progress Bars
Scrolled Views
List Views
Icon Views
Widgets for Tabular Material
Widgets for Displaying Rich Text
5. A Guided Tour Through the Qt Dialog Boxes
Predefined Dialog Boxes
File Selection Dialog Boxes
Color Dialog Boxes
Font Dialog Boxes
Message Boxes
Input Dialog Boxes
Progress Dialog Boxes
Error Message Dialog Boxes
Building Blocks for Your Own Dialog Boxes
Tab Dialog Boxes
Wizards
6. Using Layout Managers
Layout Manager Basics
Laying Out Widgets in Rows and Columns
Nested Layout Managers
Grid Layout
Implicit Geometry Management
7. Some Thoughts on GUI Design
8. Container Classes
Available Container Classes
Choosing a Container Class
Working with Reference-Based Container Classes
Basic Usage
Caching Data
Iterators
Stacks and Queues
Working with Value-Based Container Classes
9. Graphics
Animations
Printing
Managing Colors
Color Allocation
Color Models
Color Groups and Palettes
Basic QPainter: Drawing Figures
Selecting Brushes, Colors, and Pens
Drawing Operations in QPainter
Advanced QPainter
Two-Dimensional Transformations
View Transformations
Clipping
Double-Buffering and Other Nifty Techniques
Independently Movable Objects with QCanvas
Adding Different Items to the Canvas
Working with Styles
Loading and Saving Custom Image Formats
Setting a Cursor
10. Text Processing
Internationalization and Localization of On-Screen Text
Internationalizing a Qt Application
Localizing Your Application
Validating User Input
Working with Regular Expressions
Reading and Writing XML Files
Processing XML with SAX
Processing XML with DOM
Rich Text
11. Working with Files and Directories
Reading a Text File
Traversing a Directory
File Information
Reading and Writing Configuration Data
12. Interapplication Communication
Using the Clipboard
Drag-and-Drop
13. Interfacing with the Operating System
Working with Date and Time Values
Loading Code Libraries Dynamically
Spawning Child Processes
Playing Sounds
14. Writing Your Own Widgets
Implementing a Coordinate Selector
Implementing a Browse Box
15. Focus Handling
16. Advanced Event Handling
Event Filters
Sending Synthetic Events
17. Advanced Signals and Slots
Signals and Slots Revisited
Connecting Several Buttons to One Slot
Actions
18. Providing Help
19. Accessing Databases
Installation of the SQL Module
Connecting to a Database
Simple Data Retrieval
Data Retrieval with Cursors
Data Display
Data Manipulation
Anything Else?
Metadata
Transactions
More Features
20. Multithreading
Configuring Qt for Multithreading
Using Qts Multithreading Classes
Multithreading Pitfalls
Alternatives to Multithreading
21. Debugging
22. Portability
Why Portability Is Desirable
How to Write Portable Programs
Danger Ahead: When Even Qt Is Not Portable
Building Projects Portably with qmake
23. Qt Network Programming
Low-Level Socket Access
Higher-Level Network Access
24. Interfacing Qt with Other Languages and Libraries
OpenGL Programming with Qt
Writing Netscape Plug-ins
Integrating Xt Widgets
Interfacing Qt with Perl
25. Using the Visual C++ IDE for Qt Programs
Importing an Existing Makefile
Creating Your Own Project from Scratch
Using qmake to Create a Project File
Using the MS Visual Studio Integration
26. Visual Design with Qt Designer
Why Do You Need A GUI Designer?
Creating a Simple Application with the Help of Qt Designer
Becoming Familiar with Qt Designer
A first look at the property editor
A first look at the form editor
More Widgets for Our Form
Generating C++ source code from the Qt Designer file
Putting It All Together
Adding Functionality to a Dialog Box by Subclassing
Continuing Work on an Already Existing Dialog Box
Adding Functionality to the Push Buttons
Initializing the State of Widgets in the Form
Connecting Widgets to One Another
Using Layout Management
Putting a Form Together with Layouts
Popular Mechanics: Working with Spacers
Useful Techniques
Working with Signals and Slots
The connection tool
The slots tool
Changing the Tab Order
All About Layout Management
Integrating Qt Designer Files into Your Project
Using qmake for Generating and Building Qt Designer Files
Using Project Management
Creating Main Windows
Accessing Databases from Qt Designer
Accessing the Help System
Dynamically Creating Forms at Runtime
Custom Widgets
A. Answers to Exercises
Answers to Exercises in Chapter 2
Answers to Exercises in the Section Hello, world!
Answers to Exercises in the Section Using the Qt Reference Documentation
Answers to Exercises in the Section Adding an Exit Button
Answers to Exercises in the Section Introduction to Signals and Slots
Answers to Exercises in the Section Event Handling and Simple Drawings with QPainter
Answers to Exercises in Chapter 3
Answers to Exercises in the Section Adding Menus
Answers to Exercises in the Section Adding a Scrolled View
Answers to Exercises in the Section Adding a Context Menu
Answers to Exercises in the Section File I/O
Index
Colophon
SPECIAL OFFER: Upgrade this ebook with OReilly mniej
Programming with Qt. Writing Portable GUI applications on Unix and Win32. 2nd Edition (e-book) - Opinie i recenzje
Na liście znajdują się opinie, które zostały zweryfikowane (potwierdzone zakupem) i oznaczone są one zielonym znakiem Zaufanych Opinii. Opinie niezweryfikowane nie posiadają wskazanego oznaczenia.