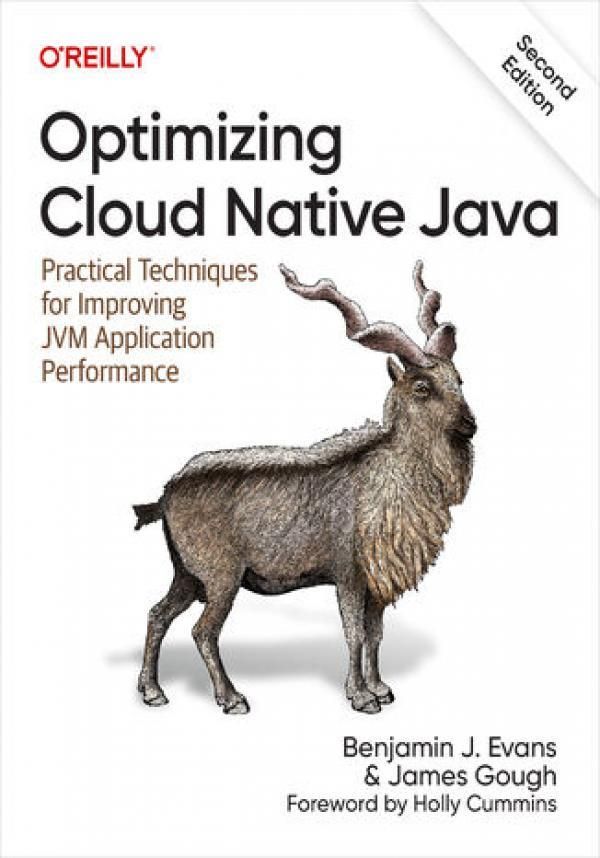
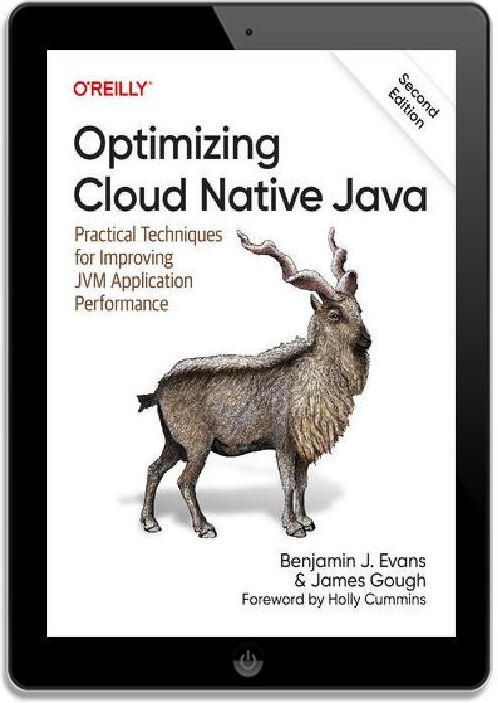
Optimizing Cloud Native Java. 2nd Edition



Optimizing Cloud Native Java. 2nd Edition - Najlepsze oferty
Optimizing Cloud Native Java. 2nd Edition - Opis
Performance tuning is an experimental science, but that doesn't mean engineers should resort to guesswork and folklore to get the job done. Yet that's often the case. With this practical book, intermediate to advanced Java technologists working with complex platforms will learn how to tune Java cloud applications for performance using a quantitative, verifiable, and repeatable approach.In response to the ubiquity of cloud computing, this updated edition of Optimizing Cloud Native Java addresses topics that are key to high performance of Java applications in the cloud. Many resources on performance tend to focus on the theory and internals of Java virtual machines, but this book discusses the low-level technical aspects within the context of performance-tuning practicalities and examines a wide range of aspects.With this book, you will:Learn how Java principles and technology make the best use of modern hardware, operating systems, and cloud stacksExamine the pitfalls of measuring Java performance numbers and the drawbacks of microbenchmarkingUnderstand how to package, deploy, operate, and debug Java/JVM applications in modern cloud environmentsApply emerging observability approaches to obtain deep understanding of cloud native applicationsUse Java language performance techniques including concurrent and distributed forms Spis treści:Foreword
Preface
Why Did We Write This Book?
Why Should You Read This Book?
Who This Book Is For
What You Will Learn
What This Book Is Not
Conventions Used in This Book
OReilly Online Learning
How to (...) więcej Contact Us
Acknowledgments
1. Optimization and Performance Defined
Java Performance the Wrong Way
Java Performance Overview
Performance as an Experimental Science
A Taxonomy for Performance
Throughput
Latency
Capacity
Utilization
Efficiency
Scalability
Degradation
Correlations Between the Observables
Reading Performance Graphs
Performance in Cloud Systems
Summary
2. Performance Testing Methodology
Types of Performance Tests
Latency Test
Throughput Test
Stress Test
Load Test
Endurance Test
Capacity Planning Test
Degradation Test
Best Practices Primer
Top-Down Performance
Creating a Test Environment
Identifying Performance Requirements
Performance Testing as Part of the SDLC
Java-Specific Issues
Causes of Performance Antipatterns
Boredom
Résumé Padding
Social Pressure
Lack of Understanding
Misunderstood/Nonexistent Problem
Statistics for JVM Performance
Types of Error
Random error
Systematic error
Non-Normal Statistics
Interpretation of Statistics
Spurious Correlation
The Hat/Elephant Problem
Cognitive Biases and Performance Testing
Reductionist Thinking
Confirmation Bias
Fog of War (Action Bias)
Risk Bias
Summary
3. Overview of the JVM
Interpreting and Classloading
Executing Bytecode
Introducing HotSpot
Introducing Just-in-Time Compilation
JVM Memory Management
Threading and the Java Memory Model
Monitoring and Tooling for the JVM
Java Implementations, Distributions, and Releases
Choosing a Distribution
The Java Release Cycle
Summary
4. Understanding Garbage Collection
Introducing Mark and Sweep
Garbage Collection Glossary
Introducing the HotSpot Runtime
Representing Objects at Runtime
GC Roots
Allocation and Lifetime
Weak Generational Hypothesis
Production GC Techniques in HotSpot
Thread-Local Allocation
Hemispheric Collection
The Classic HotSpot Heap
The Parallel Collectors
Young Parallel Collections
Old Parallel Collections
Serial and SerialOld
Limitations of Parallel Collectors
The Role of Allocation
Summary
5. Advanced Garbage Collection
Tradeoffs and Pluggable Collectors
Concurrent GC Theory
JVM Safepoints
Tri-Color Marking
Forwarding Pointers
G1
G1 Heap Layout and Regions
G1 Collections
G1 Mixed Collections
Remembered Sets
Full Collections
JVM Config Flags for G1
Shenandoah
Concurrent Evacuation
JVM Config Flags for Shenandoah
Shenandoahs Evolution
ZGC
Balanced (Eclipse OpenJ9)
OpenJ9 Object Headers
Large Arrays in Balanced
NUMA and Balanced
Niche HotSpot Collectors
CMS
Epsilon
Summary
6. Code Execution on the JVM
Lifecycle of a Traditional Java Application
Overview of Bytecode Interpretation
Introduction to JVM Bytecode
Simple Interpreters
HotSpot-Specific Details
JIT Compilation in HotSpot
Profile-Guided Optimization
Klass Words, Vtables, and Pointer Swizzling
Compilers Within HotSpot
The Code Cache
Logging JIT Compilation
Simple JIT Tuning
Evolving Java Program Execution
Ahead-of-Time (AOT) Compilation
Quarkus
GraalVM
Summary
7. Hardware and Operating Systems
Introduction to Modern Hardware
Memory
Memory Caches
Modern Processor Features
Translation Lookaside Buffer
Branch Prediction and Speculative Execution
Hardware Memory Models
Operating Systems
The Scheduler
The JVM and the Operating System
Context Switches
A Simple System Model
Utilizing the CPU
Garbage Collection
I/O
Mechanical Sympathy
Summary
8. Components of the Cloud Stack
Java Standards for the Cloud Stack
Cloud Native Computing Foundation
Kubernetes
Prometheus
OpenTelemetry
Virtualization
Choosing the Right Virtual Machines
Virtualization Considerations
Images and Containers
Image Structure
Building Images
Running Containers
Networking
Introducing the Fighting Animals Example
Summary
9. Deploying Java in the Cloud
Working Locally with Containers
Docker Compose
Tilt
Container Orchestration
Deployments
Sharing Within Pods
Container and Pod Lifecycles
Services
Connecting to Services on the Cluster
Challenges with Containers and Scheduling
Working with Remote Containers Using Remocal Development
Deployment Techniques
Blue/Green Deployments
Canary Deployments
Evolutionary Architecture and Feature Flagging
Java-Specific Concerns
Containers and GC
Memory and OOMEs
Summary
10. Introduction to Observability
The What and the Why of Observability
What Is Observability?
Why Observability?
The Three Pillars
Metrics
Logs
Traces
The Pillars as Data Sources
ProfilingA Fourth Pillar?
Observability Architecture Patterns and Antipatterns
Architectural Patterns for Metrics
Manual Versus Automatic Instrumentation
Antipattern: Shoehorn Data into Metrics
Antipattern: Abusing Correlated Logs
Diagnosing Application Problems Using Observability
Performance Regressions
Unstable Components
Repartitioning and Split-Brain
Thundering Herd
Cascading Failure
Compound Failures
Vendor or OSS Solution?
Summary
11. Implementing Observability in Java
Introducing Micrometer
Meters and Registries
Counters
Gauges
Meter Filters
Timers
Distribution Summaries
Runtime Metrics
Introducing Prometheus for Java Developers
Prometheus Architecture Overview
Using Prometheus with Micrometer
Introducing OpenTelemetry
What Is OpenTelemetry?
Why Choose OTel?
OTLP
The Collector
OpenTelemetry Tracing in Java
Manual Tracing
Automatic Tracing
Sampling Traces
OpenTelemetry Metrics in Java
OpenTelemetry Logs in Java
Summary
12. Profiling
Introduction to Profiling
GUI Profiling Tools
VisualVM
JDK Mission Control
Sampling and Safepointing Bias
Modern Profilers
perf
Async Profiler
JDK Flight Recorder (JFR)
Operational Aspects of Profiling
Using JFR As an Operational Tool
Red Hat Cryostat
JFR and OTel Profiling
Choosing a Profiler
Memory Profiling
Allocation Profiling
Heap Dumps
Summary
13. Concurrent Performance Techniques
Introduction to Parallelism
Amdahls Law
Fundamental Java Concurrency
Understanding the JMM
Building Concurrency Libraries
Method and Var Handles
Atomics and CAS
Locks and Spinlocks
Summary of Concurrent Libraries
Locks in java.util.concurrent
Read/Write Locks
Semaphores
Concurrent Collections
Latches and Barriers
Executors and Task Abstraction
Introducing Asynchronous Execution
Selecting an ExecutorService
Fork/Join and Parallel Streams
Actor-Based Techniques
Virtual Threads
Introduction to Virtual Threads
Virtual Thread Concurrency Patterns
Summary
14. Distributed Systems Techniques
and Patterns
Basic Distributed Data Structures
Clocks, IDs, and Write-Ahead Logs
Two-Phase Commit
Object Serialization
Data Partitioning and Replication
CAP Theorem
Consensus Protocols
Paxos
Raft
Distributed Systems Examples
Distributed DatabaseCassandra
In-Memory Data GridInfinispan
Event StreamingKafka
Enhancing Fighting Animals
Introducing Kafka to Fighting Animals
A Simple Hospital Service
An Active Hospital
Summary
15. Modern Performance and The Future
New Concurrency Patterns
Structured Concurrency
Scoped Values
Panama
Leyden
Images, Constraints, and Condensers
Leyden Premain
Valhalla
Conclusions
A. Microbenchmarking
Introduction to Measuring Low-Level Java Performance
Introduction to JMH
Dont Microbenchmark If You Can Help It (A True Story)
Heuristics for When to Microbenchmark
The JMH Framework
Executing Benchmarks
B. Performance Antipatterns Catalog
Distracted by Shiny
Example Comments
Reality
Discussion
Resolutions
Distracted by Simple
Example Comments
Reality
Discussion
Resolutions
Performance Tuning Wizard
Example Comment
Reality
Discussion
Resolutions
Tuning by Folklore
Example Comment
Reality
Discussion
Resolutions
The Blame Donkey
Example Comment
Reality
Discussion
Resolutions
Missing the Bigger Picture
Example Comments
Reality
Discussion
Resolutions
UAT Is My Desktop
Example Comment
Reality
Discussion
Resolutions
Production-Like Data Is Hard
Example Comments
Reality
Discussion
Resolutions
Index mniej
Optimizing Cloud Native Java. 2nd Edition - Opinie i recenzje
Na liście znajdują się opinie, które zostały zweryfikowane (potwierdzone zakupem) i oznaczone są one zielonym znakiem Zaufanych Opinii. Opinie niezweryfikowane nie posiadają wskazanego oznaczenia.